Rich Messaging Developer Guide
For non technical information on how to build rich messaging elements in Quiq, refer to Message Catalog, Snippets, or the Bot designer guide
Overview
The messaging space offers many different platforms that can be used to engage with customers, including SMS, Web Chat, Apple Messages, RCS Business Messaging, Whatsapp, Instagram, Facebook Messenger and more. All of these platforms have different APIs and each API takes a different set of messaging objects that are all formatted specific to each API. For example, SMS supports text and images, while Apple Messages allows for selecting from lists and pushing apps
SMS and Google RBM:
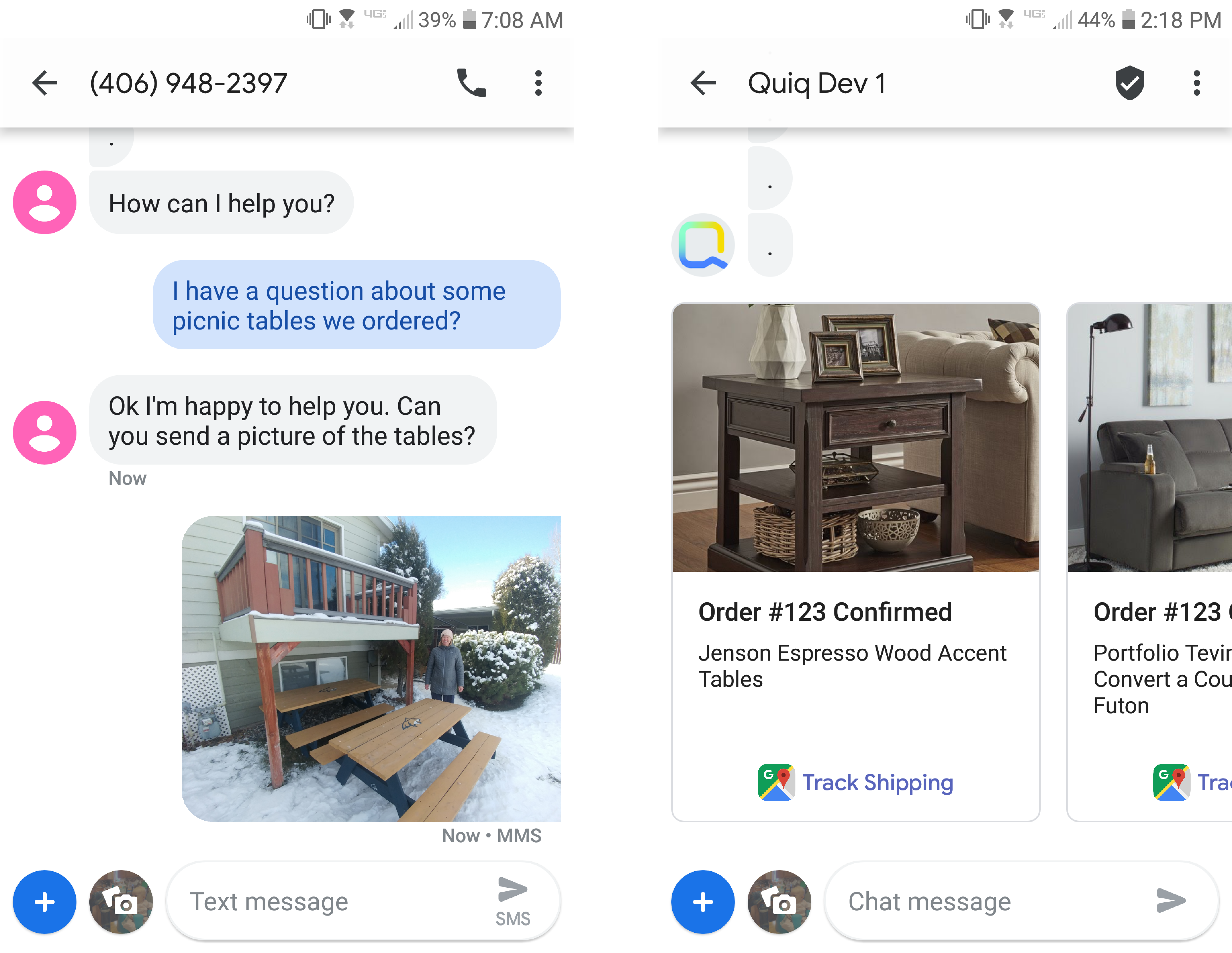
The Apple Selection List:
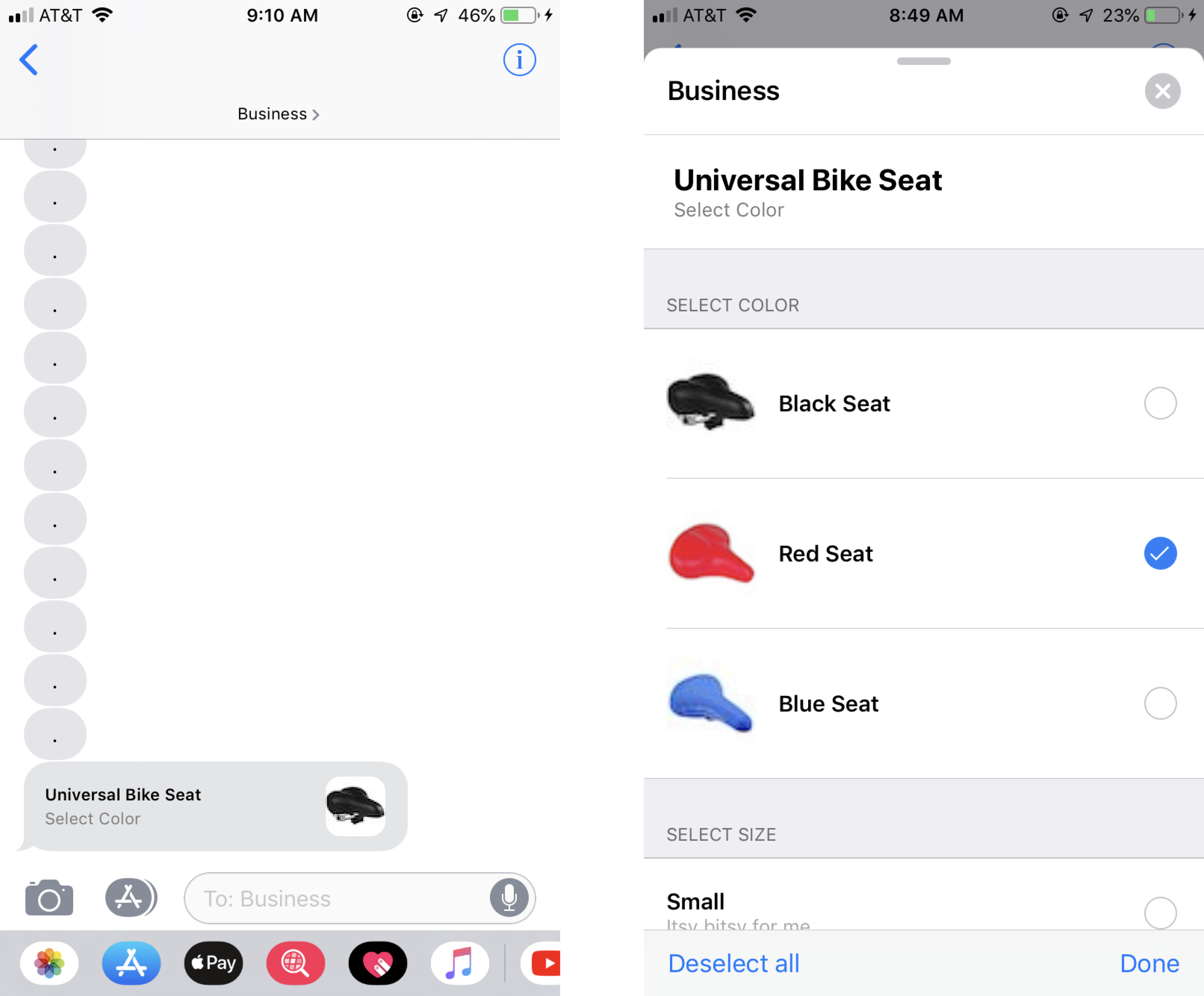
As a developer, providing a set of messaging capabilities across all of these platforms is a daunting task. You need to know about the different protocols and APIs of each one, as well as what types of messages it is able to present to users. It does not take long for the cost of creating and maintaining messaging solutions across these platforms to become very expensive and error prone.
Wouldnât it be great if you could create one set of messages that can work across all of these platforms, and at the same time deliver an experience for users that utilizes the individual power of each messaging platform?
Quiq's Conversational Engagement Platform solution features a messaging abstraction that allows you to leverage the power of the interactions offered by all of Quiq's family of supported platforms, without having to invest the time to build and manage separate messages for each individual platform. Quiq currently supports the following platforms, for more detailed information on each platform, refer to Channels:
- SMS
- Web Chat
- Apple Messages
- RCS Business Messaging
- Facebook Messenger
- X (formerly known as Twitter)
Additionally, the Quiq Conversational Engagement Platform solution allows you to tie actions to the responses users provide to the messages you send them. These include the ability to capture data from the response and bind it to the conversation or customer records, and also the ability to route conversations to queues, or different locations in your AI assistant based on responses. These capabilities allow you to build workflows to help guide users to the right resolution efficiently.
Abstractions
In this guide we will focus on Quiqâs messaging abstractions, called Quiq Abstractions, which allow you to build messages that take advantage of a platformâs most unique and powerful offerings, while at the same time remaining compatible across all other messaging platforms. Specifically, we will be covering:
- Quiq Replies: Send a message or question with a list of possible responses for a user to choose from.
- Times: Send a message or question with a list of possible times for a user to choose from.
- Cards: Send an image along with text headers and buttons.
- Carousels: Send one or multiple carousel cards. Carousel cards can contain images, text, subtitles, titles, side effects, postback actions, urls, and reply directives.
- Authentication: Send a user an authentication request, which allows them to sign in and verify their identity. The results of this operation are shown to agents/bots, who can use the information accordingly.
- Pay: Securely send payment requests and receive payments from your users.
- Proxy Interactions: Send a platform specific interaction. This allows you to leverage the full capabilities of a platform when you want to do something really snazzy that isn't supported by other platforms.
- Reply Directives: Bind data and workflow actions to possible user responses on your message.
- Combining Messages: Combine Quiq Abstractions to create richer, more powerful messages and interactions with users.
These messaging abstractions are used across some of Quiqâs public API that support bots, sending notifications, and starting conversations, and are referenced frequently in the guides for these areas.
Throughout this guide we will show example images of what the different forms of messages will look like on the following platforms: Apple Messages, Google RBM, Webchat, and SMS.
The following resources are referenced frequently by this guide:
Quiq Replies
The first type of Quiq Abstraction we will be covering is Quiq Replies. This abstraction allows you to send a message or question, along with a list of possible responses. For example, you could send the following question to help determine what kind of help a user requires:
âHow can I help you today? Service Request, Product Question, Account Infoâ
While you can send this message as a text snippet and the user can manually type in a response, wouldnât it be faster if the user was presented with a list of options and they could either tap them, or at least just respond with the first letter of one of the options? With Quiq Replies these kinds of things are a snap.
To do so you would build your message in the following way:
{
"text": "How can I help you today?",
"quiqReply": {
"replies": [{
"text": "Account Info",
},
{
"text": "Service Request",
},
{
"text": "Product Question",
}
]
}
}
Because you are using the quiqReply Quiq Abstraction, you are guaranteed support across all platforms, so you donât need to create a different message for Apple, Google, Web chat and SMS. It will just work! For Google, Apple and Web chat the user will see their rich, native implementation of this type of message, and for SMS they will see a nicely formatted textual representation that allows the user to select an option by typing a single character.
Quiq Replies on Google RBM, Web chat and SMS:
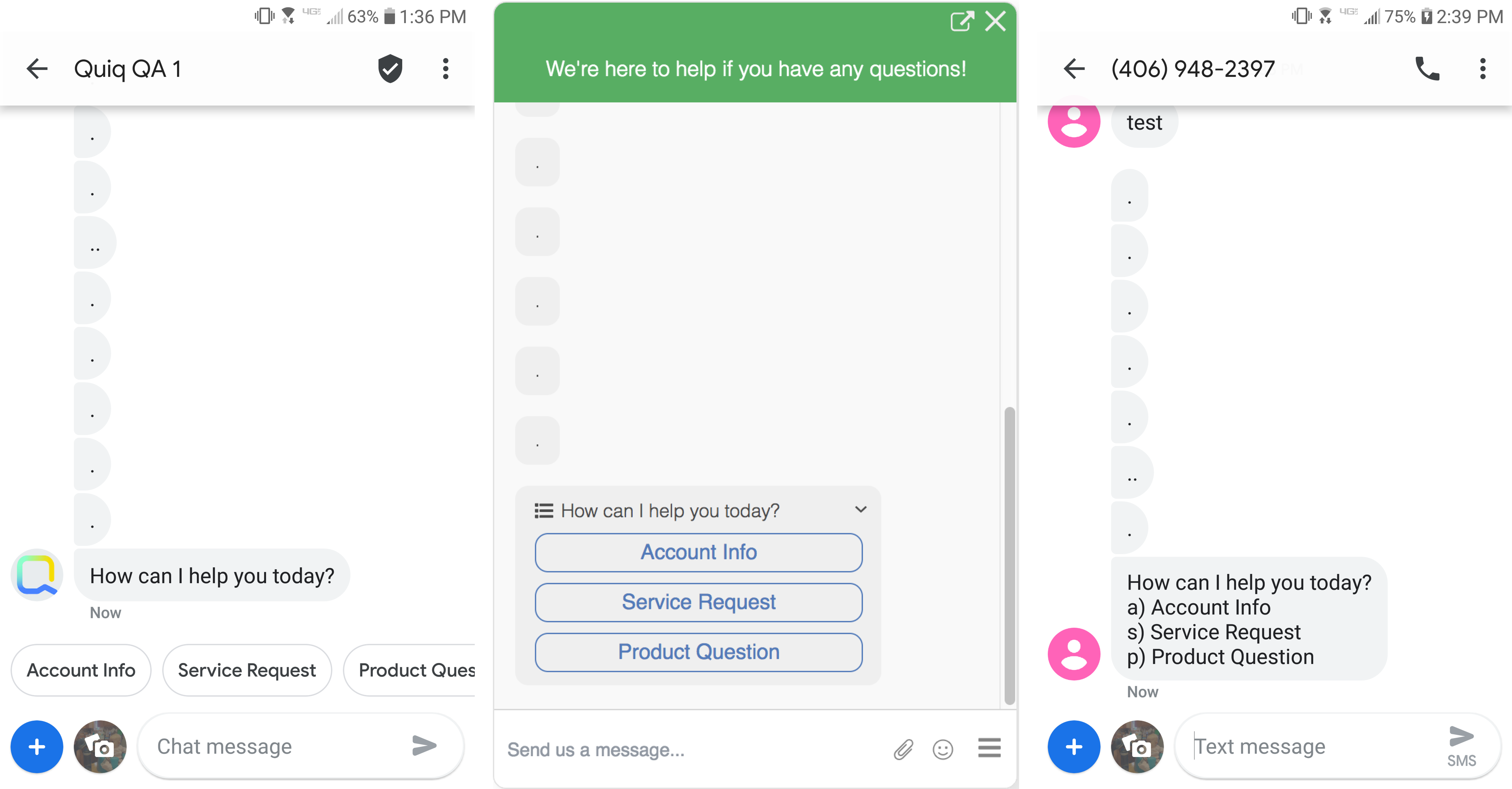
Quiq Replies on Apple Messages:
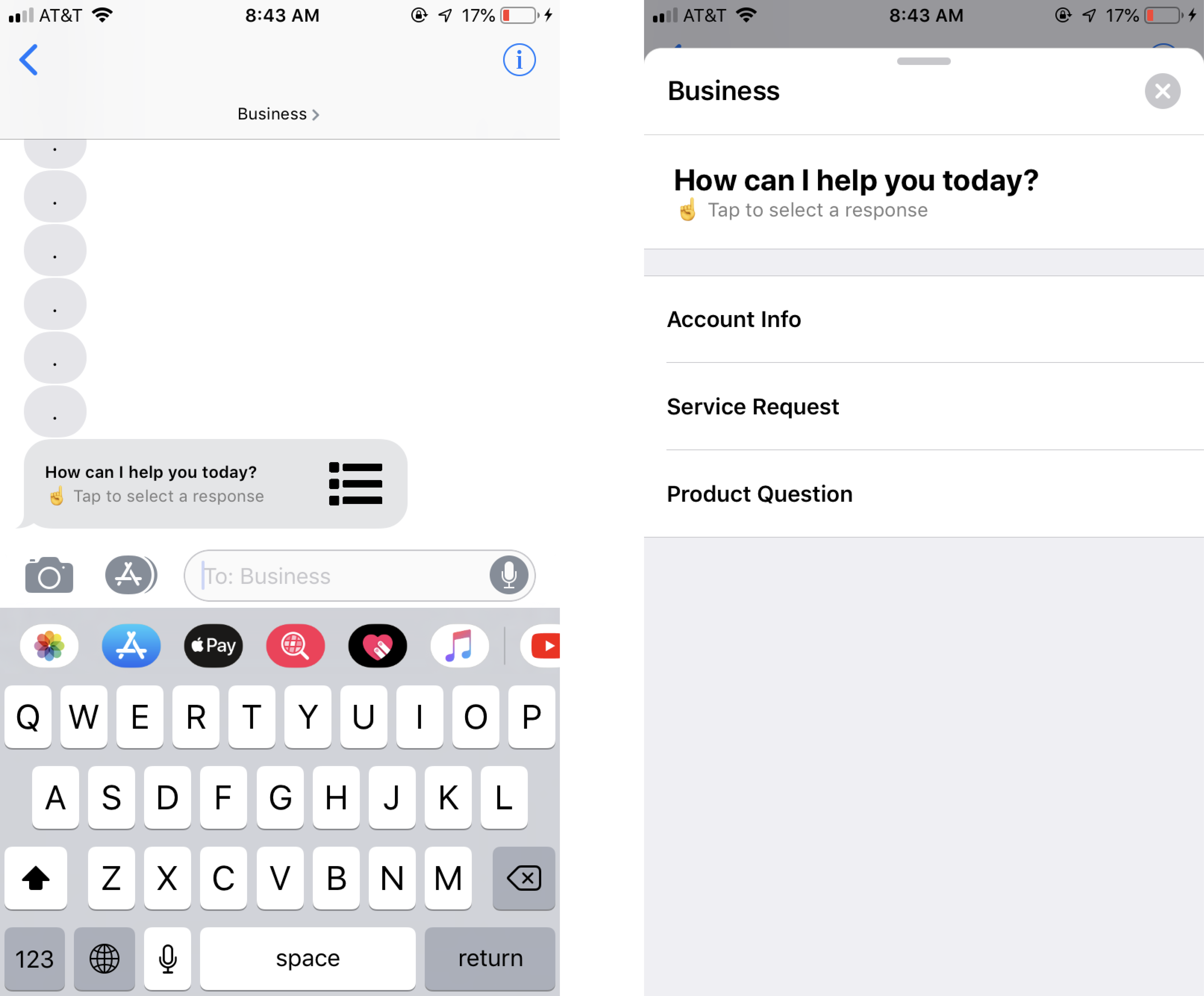
Times
Time interactions are similar to Quiq Replies, and can be used to handle scheduling requests. For example, if you wanted to ask a user to pick a time to come in for a service appointment you would do the following:
{
"text": "Please select a time to come in for service.",
"times": {
"items": [{
"startTime": <STARTING_TIMESTAMP_IN_MILLISECONDS>,
âdurationâ: 1800,
},
{
"startTime": <STARTING_TIMESTAMP_IN_MILLISECONDS>,
âdurationâ: 1800,
},
{
"startTime": <STARTING_TIMESTAMP_IN_MILLISECONDS>,
âdurationâ: 1800,
},
]
}
}
With this type of interaction, the user will be presented with 3 time based options. When they select one, the agent will see the response, and if the platform supports it, an appointment will be made in the userâs calendar. But again, because you are using a Quiq Abstraction, support across all platforms is guaranteed.
Times on Google RBM, Webchat and SMS:
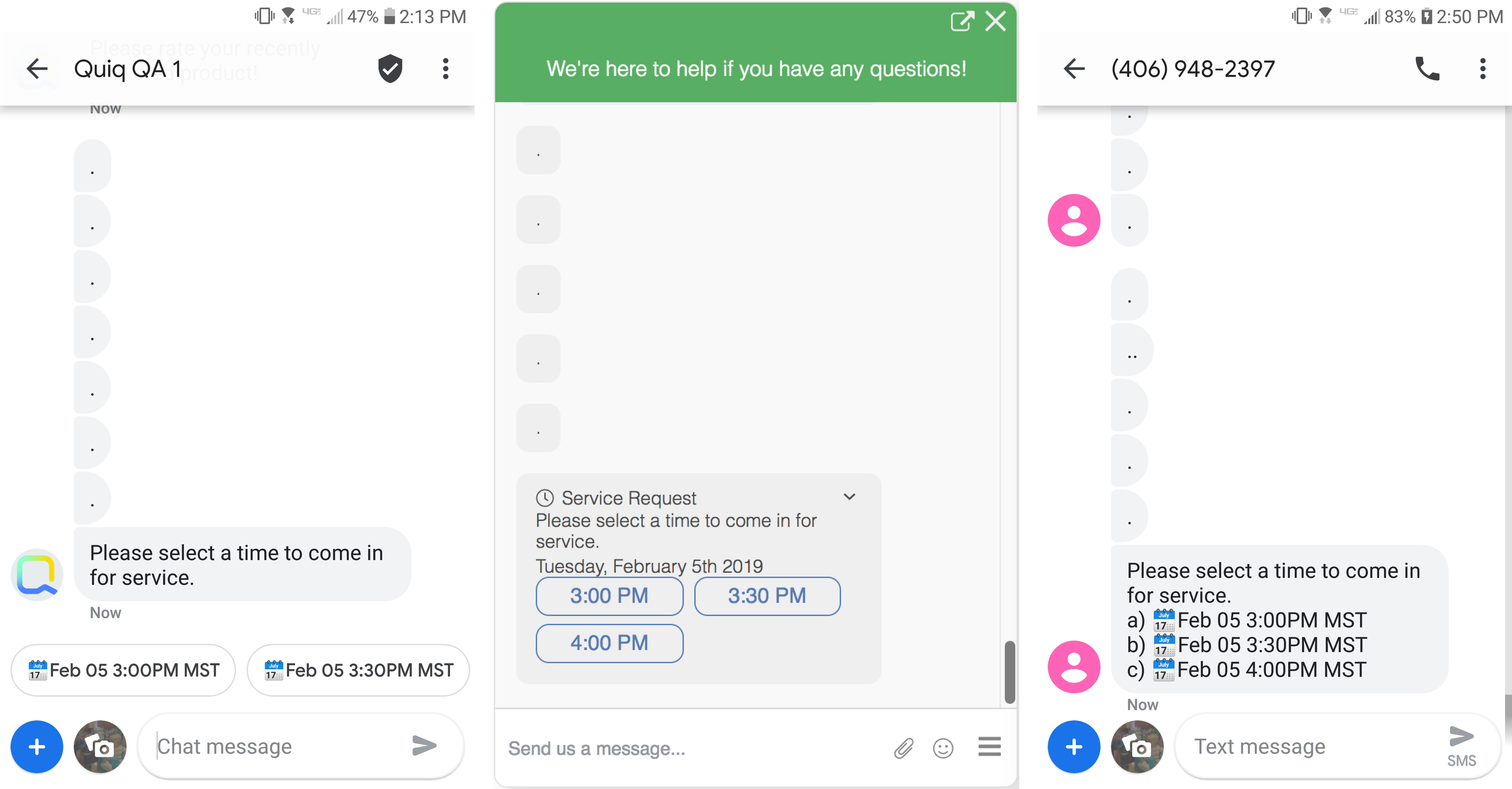
Times on Apple Messages:
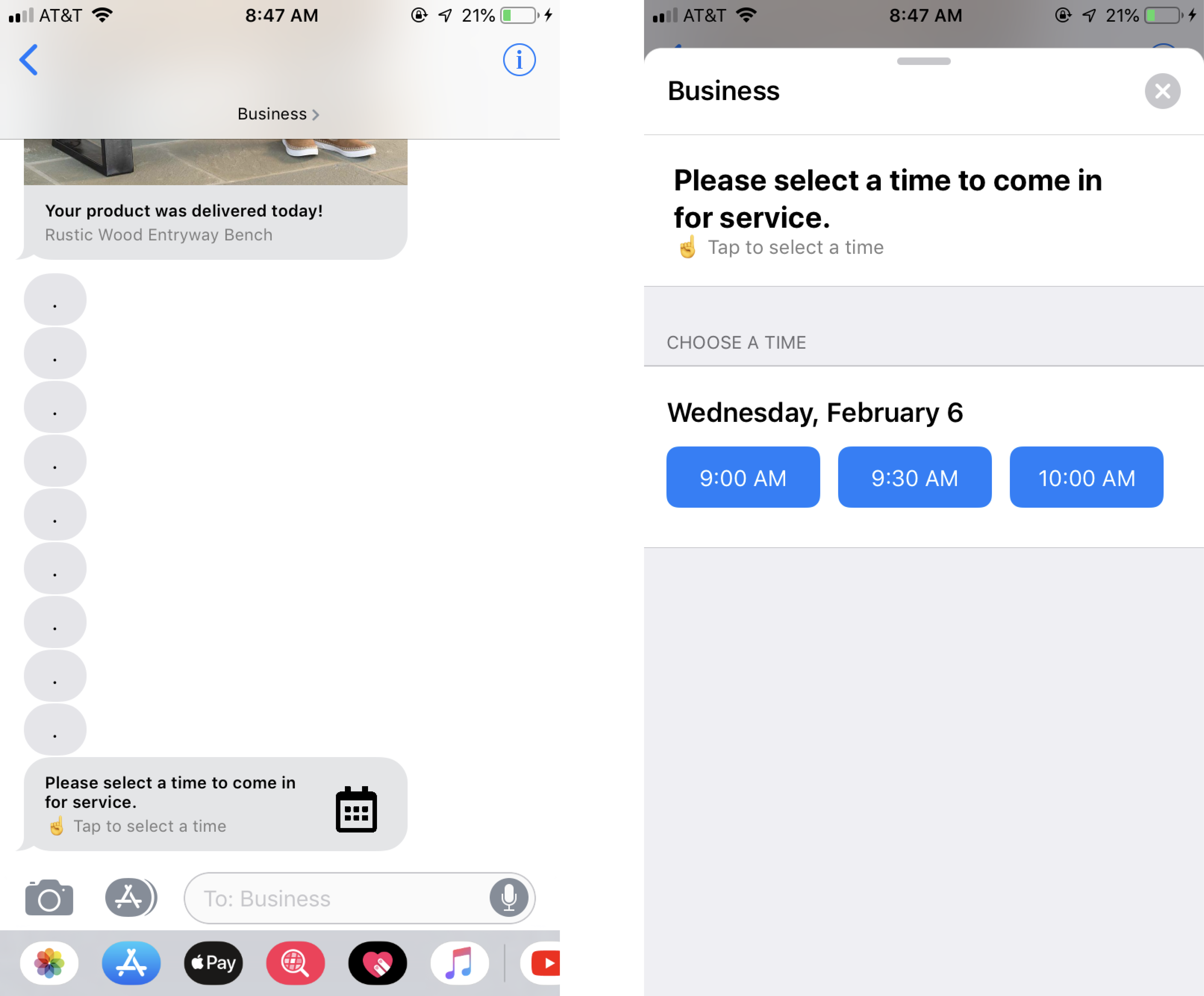
Cards
The Card Quiq Abstraction is a way to send out a message that contains an image, text, and a link all bundled together. This can be useful when sending out product offers, delivery notifications, or appointment reminders, just to name a few scenarios. In this example we show how to implement a simple product special.
{
"card": {
"title": âPlease check out our weekly product special!â,
âsubTitleâ: âThis is exclusive to our members!â,
âimageâ: {
âpublicUrlâ: âhttp\://urlToPublicImageâ
},
âlinkâ: {
âurlâ: âhttps\://linktonlinestoreâ
}
}
}
This abstraction allows you to specify a publicly accessible image, and also a link url that will be used if the user taps on the card. There are also several title options to choose from as well. For a complete listing please see the public API documentation.
Google RBM, Webchat and SMS:
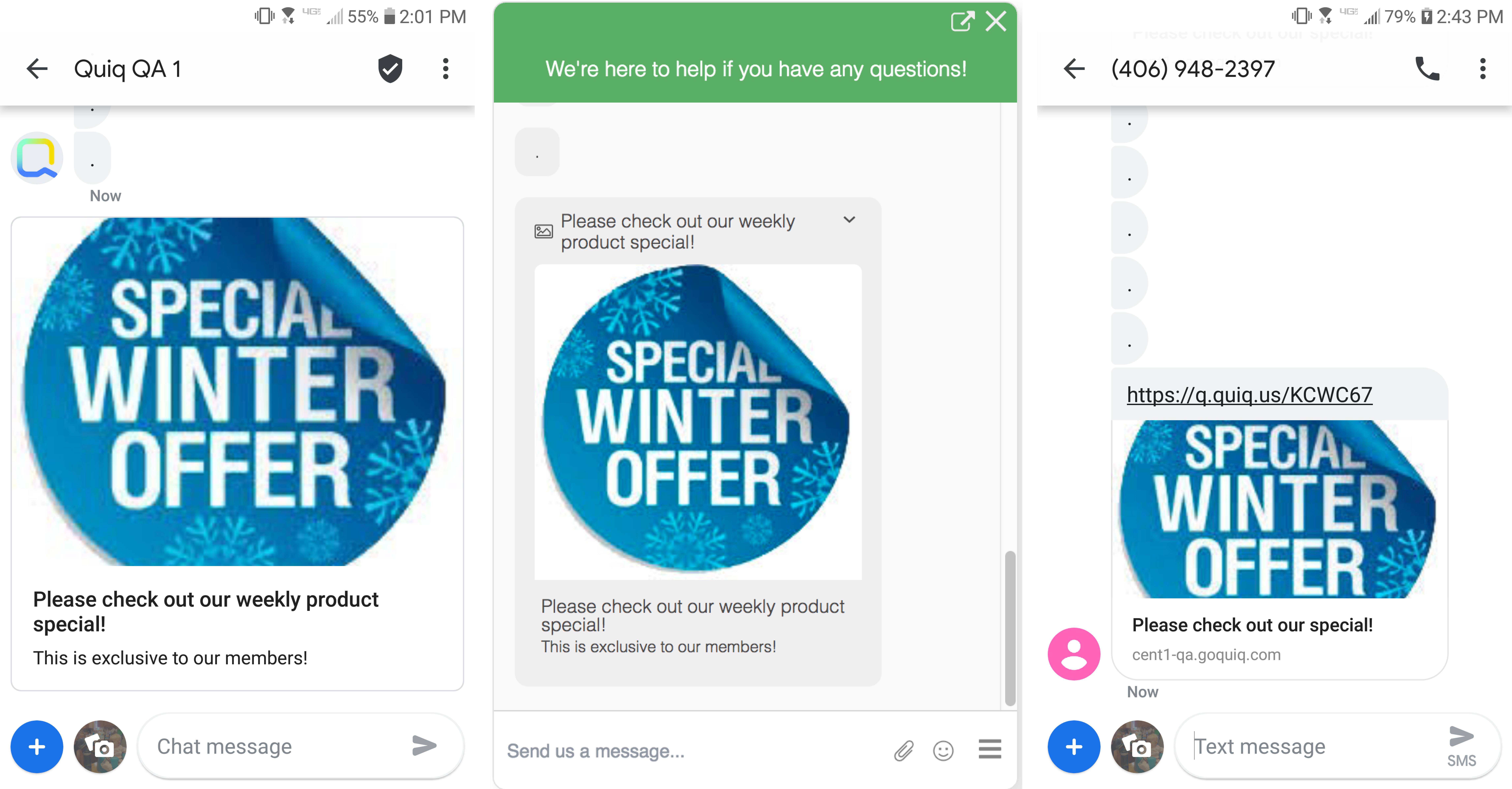
Apple Messages:
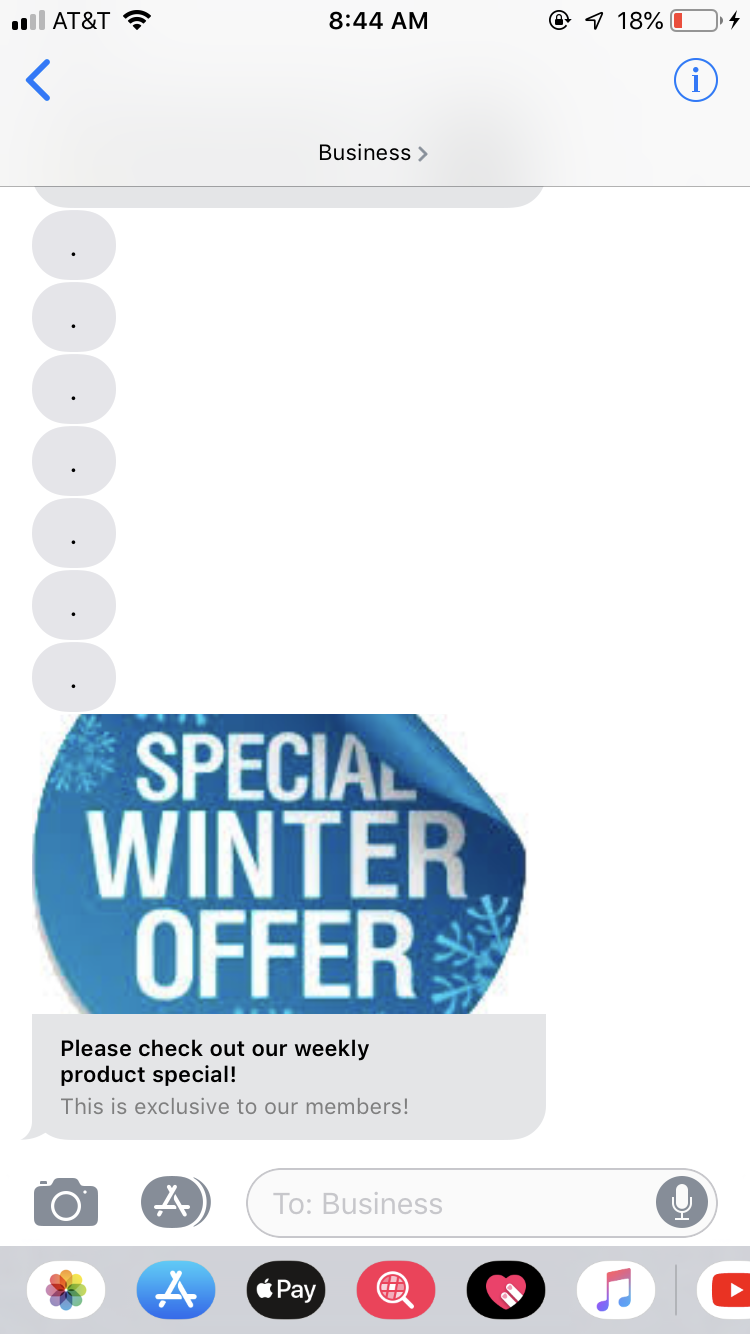
Note that while Apple, Google and Web chat all support rich implementations of the cards, SMS is more limited. In any situation where a platform is not able to natively handle a message, we fall back to the Quiq Interaction App, which is displayed on the platform as a shortened link (and when possible with an Open Graph preview). The user can click on this link to see the full message, and then either tap a button to return to the channel, or else when they make a selection they will be taken back automatically. The Quiq Interaction App is how the Quiq Conversational Engagement Platform guarantees a good user experience for Quiq Abstractions on platforms like SMS, regardless of how complex your message is. We will see more examples of this later in this guide.
Carousels
The Carousel abstraction is a way to send out one or multiple cards, consisting of a combination of an image, a title, a subtitle, a url, or buttons - buttons can either go to a location within a bot via a postbackAction, or go to an external url. You can send multiple carousel cards at a time, although different platforms have different limitations on the number of carousels that can be sent at once.
In this example weâll see how we can send a greeting menu consisting of an initial message and 3 carousel cards, each with an image, a title, and a button that send users down specific flows in the AI Assistant:
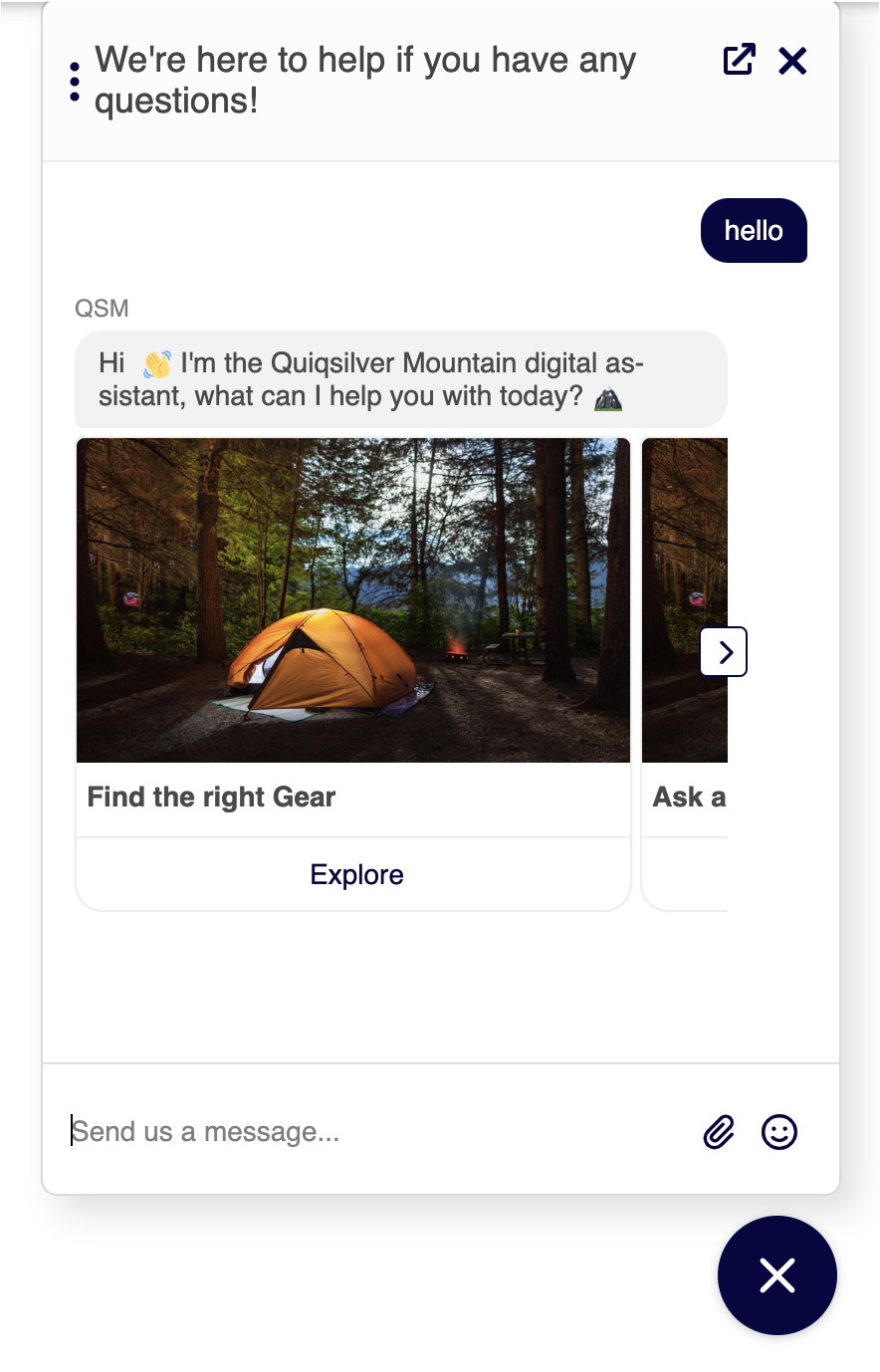
{
"text": "\"Hi đ I'm the Quiqsilver Mountain digital assistant, what can I help you with today? đ",
"assets": [],
"carousel": {
"cardWidth": null,
"cards": [
{
"image": {
"publicUrl": "https://quiqsilvermountain.com/cdn/shop/files/camping_gear_720x.jpg?v=1622218822"
},
"buttons": [
{
"text": "Explore"
}
],
"subTitle": "",
"link": {
"url": ""
},
"title": "Find the right Gear"
},
{
"image": {
"publicUrl": "https://quiqsilvermountain.com/cdn/shop/files/image_3_360x.png?v=1622223062"
},
"buttons": [
{
"text": "Ask"
}
],
"subTitle": "",
"link": {
"url": ""
},
"title": "Ask a Question"
},
{
"image": {
"publicUrl": "https://quiqsilvermountain.com/cdn/shop/files/image_2_360x.png?v=1622222948"
},
"buttons": [
{
"text": "Speak to a rep"
}
],
"subTitle": "",
"link": {
"url": ""
},
"title": "Request Help"
}
],
"selectable": false,
"suppressFreeTextResponses": false,
"displayPreferences": {
"cardLayoutDirection": "Horizontal"
}
}
}
You can set cardLayoutDrection
to either Horizontal
or Vertical
to change the orientation of your cards:
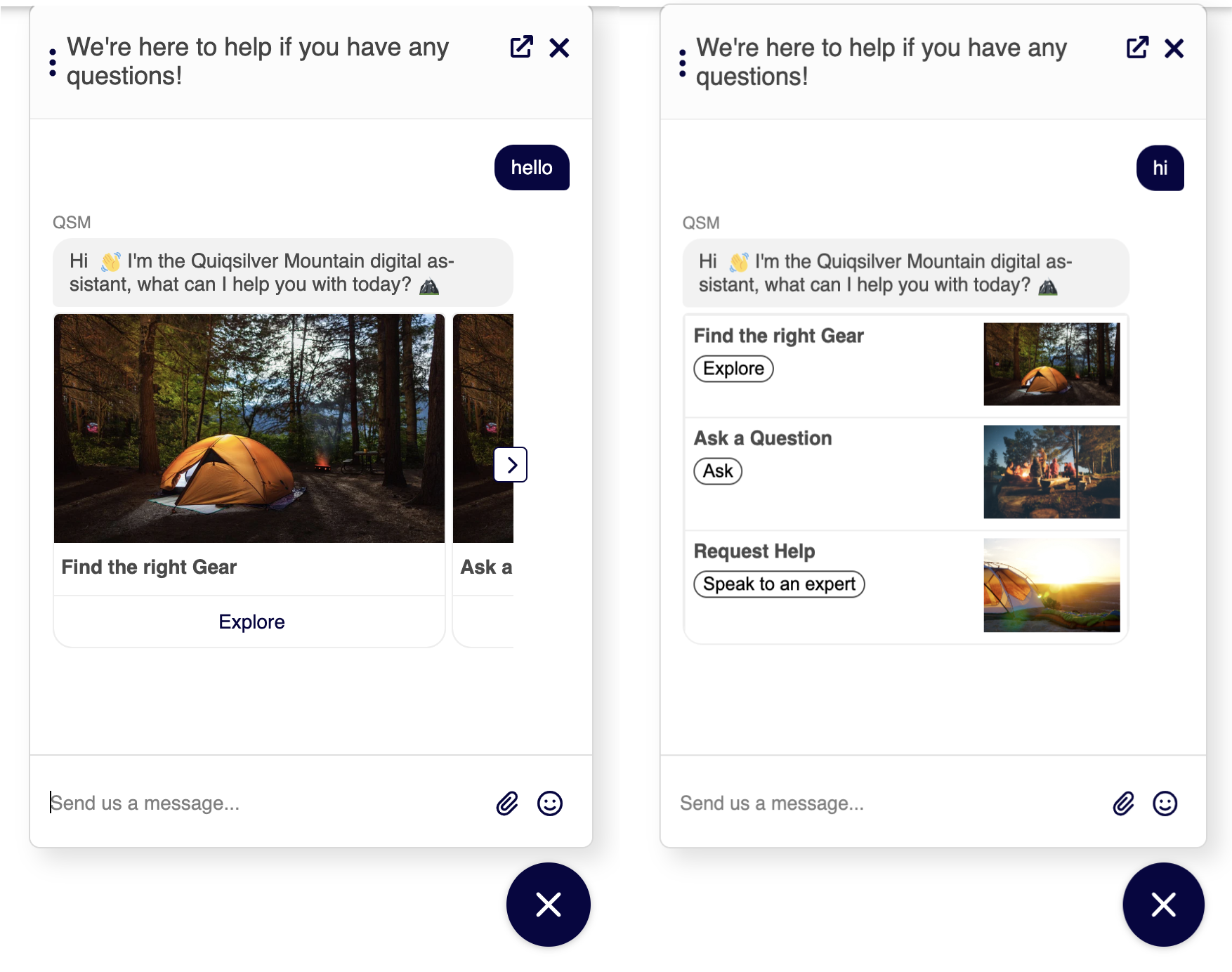
In next example weâll build a product card with a button that transfers to a sales queue, and a button that takes the customer to the web page for the tent theyâre looking at:
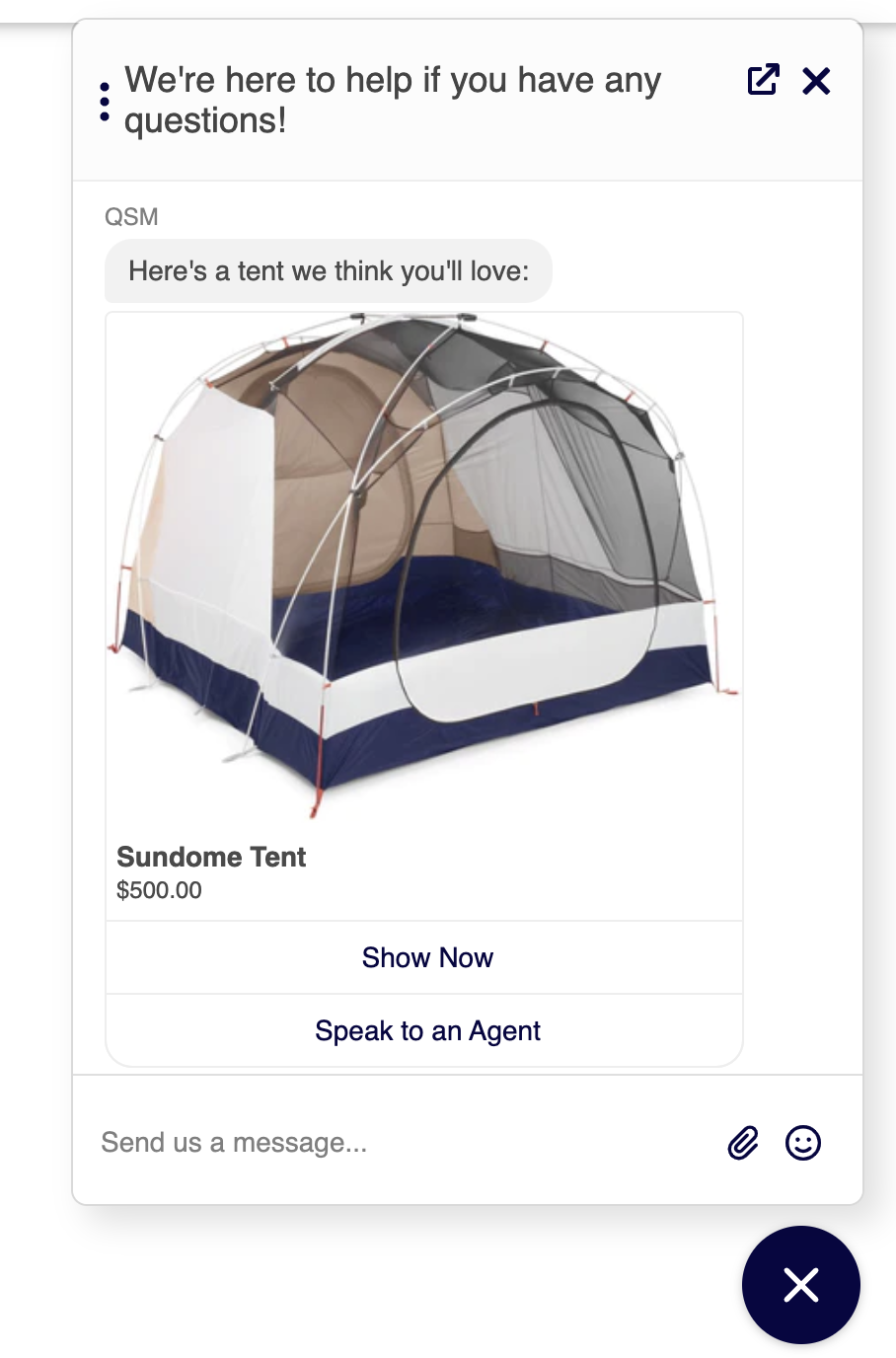
{
"text": "Here's a tent we think you'll love:",
"assets": [],
"carousel": {
"cardWidth": null,
"cards": [
{
"image": {
"publicUrl": "https://quiqsilvermountain.com/cdn/shop/products/sundometent_360x.jpg?v=1622220785"
},
"buttons": [
{
"text": "Shop Now",
"action": {
"type": "openUrlAction",
"url": "https://quiqsilvermountain.com/collections/tents/products/sundome-tent",
"target": "New"
}
},
{
"text": "Speak to an Agent"
}
],
"subTitle": "$500.00",
"link": {
"url": "https://quiqsilvermountain.com/collections/tents/products/sundome-tent"
},
"title": "Sundome Tent"
}
],
"selectable": false,
"suppressFreeTextResponses": false,
"displayPreferences": {
"cardLayoutDirection": "Horizontal"
}
}
}
Notes:
- When sending a carousel card, you must also send a message along with it.
- At the time of writing, buttons on carousels are not fully supported on all messaging platforms, please test ahead of time to ensure your buttons are behaving as expected.
- Only one of the following is required to create a carousel card, although a card can contain all of these in tandem:
A title, a subtitle, a URL, a button, or an image - Horizontal and vertical carousel card orientations are not supported on all messaging channels outside of web chat. Quiq will do itâs best to fallback to the supported visualization for the channel youâre on, but ensure you test how your card renders on the channel you intend to deploy on.
- A carousel card can have a maximum of 3 buttons.
- The maximum number of carousel cards varies depending on the channel youâre sending on, for web chat, Quiq supports a default of 10 carousel cards in one carousel.
- When using a URL button in your card, you can specify the target for your URL as New, Same, or Webview. This will work as expected on web chat, but will not on other channels, like SMS.
Authentication
Authentication Quiq Abstractions allow users to identify themselves so that agents can be sure they are dealing with the actual user, and also to prevent agents from having to ask repetitive questions about the user. To do this you would create a message like the following (you would work with us to register your Oauth provider and get a configurationId):
{
"auth": {
"configurationId": <Oath Configuration Id>,
"failureMessage": "Failed Authorization",
"identity": null,
"successMessage": "Successfully Authorized"
},
"text": "Please login to confirm your identity",
}
The above interaction would be rendered in the following way across in Apple Messages:
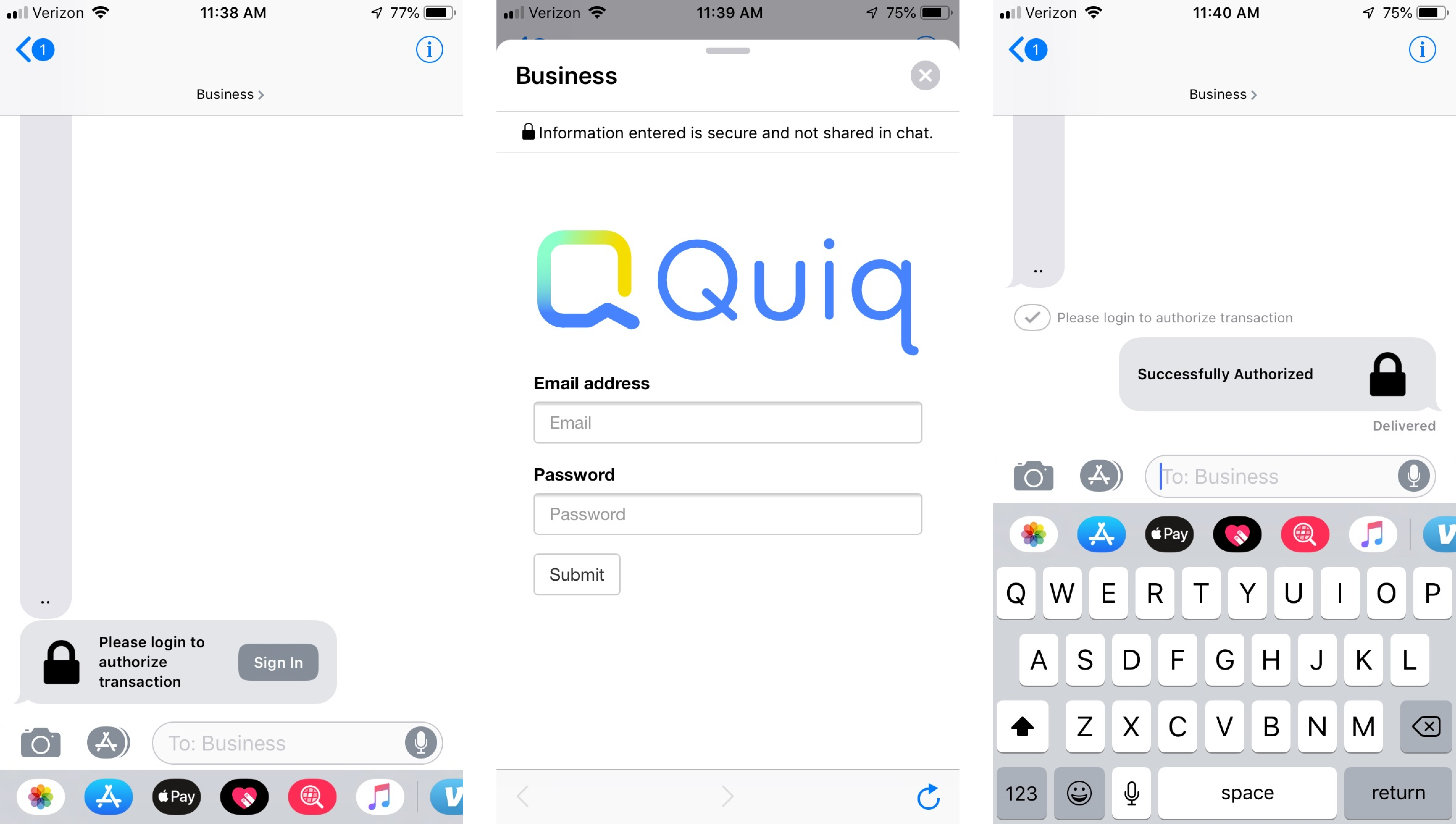
It would be rendered like this in Web chat:
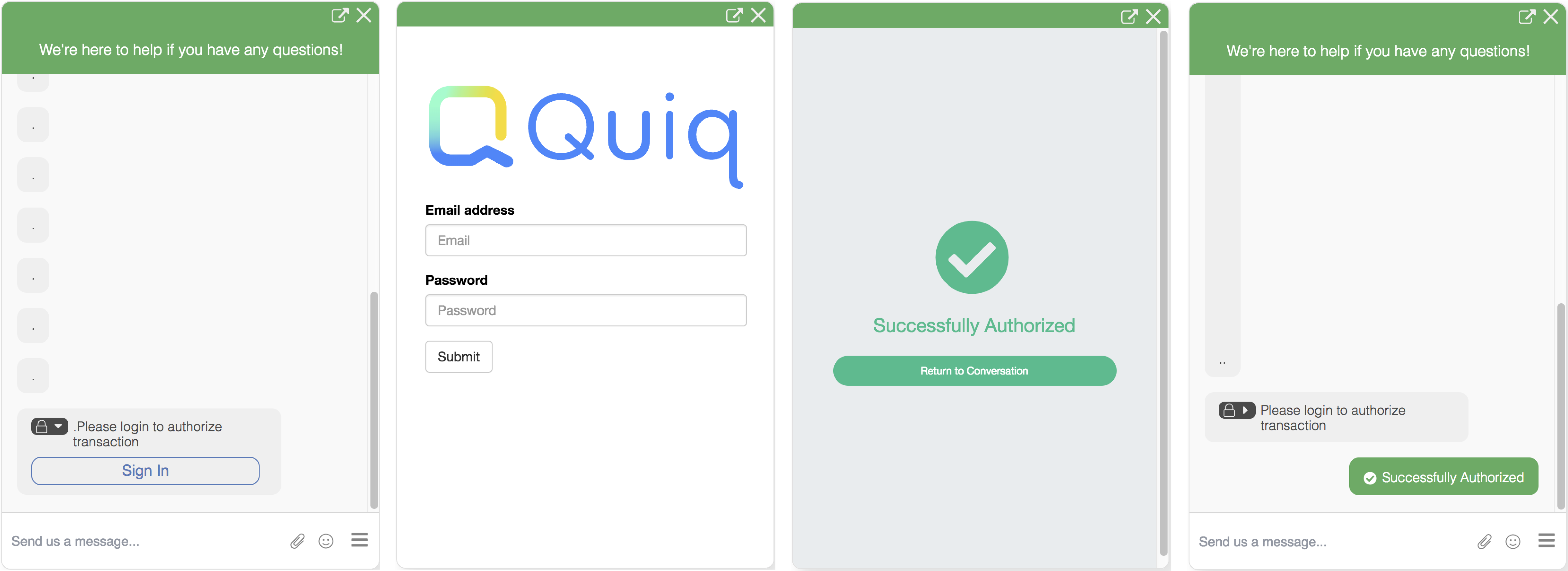
In the Apple Messages and Web chat case, we have a native implementation of authorization being used, while in Google and SMS, the message is again handled via the Quiq Interaction App, which allows the user to perform the authorization before returning to the channel. In all cases the user remains within a secure environment the entire time.
Pay
Quiq offers a native pay integration that enables the collection of payments via a variety of methods such as credit card entry and Apple Pay. This integration will have to first be enabled for your tenant, and you must ensure youâve completed the initial setup process outlined in the Quiq Pay Integration documentation.
In this example, weâre sending an invoice to a user interested in purchasing trip cancellation insurance for an upcoming whitewater rafting trip:
{
"text": "Here's your invoice for your whitewater rafting cancellation insurance:",
"assets": [],
"pay": {
"currencyCode": "USD",
"total": {
"label": "Total",
"amount": 4599
},
"lineItems": [
{
"label": "Trip Insurance",
"amount": 4599
}
],
"description": "Quiqsilver Mountain Rafting Trip Cancellation Insurance",
"processorId": "brain-tree"
},
"transcriptHints": {
"heading1": "Here's your invoice for your whitewater rafting cancellation insurance:",
"heading2": "",
"description": "Payment request",
"icon": {
"builtInIcon": "payment"
}
}
}
Hereâs how the pay message comes across in Conversation Starter Web Chat:
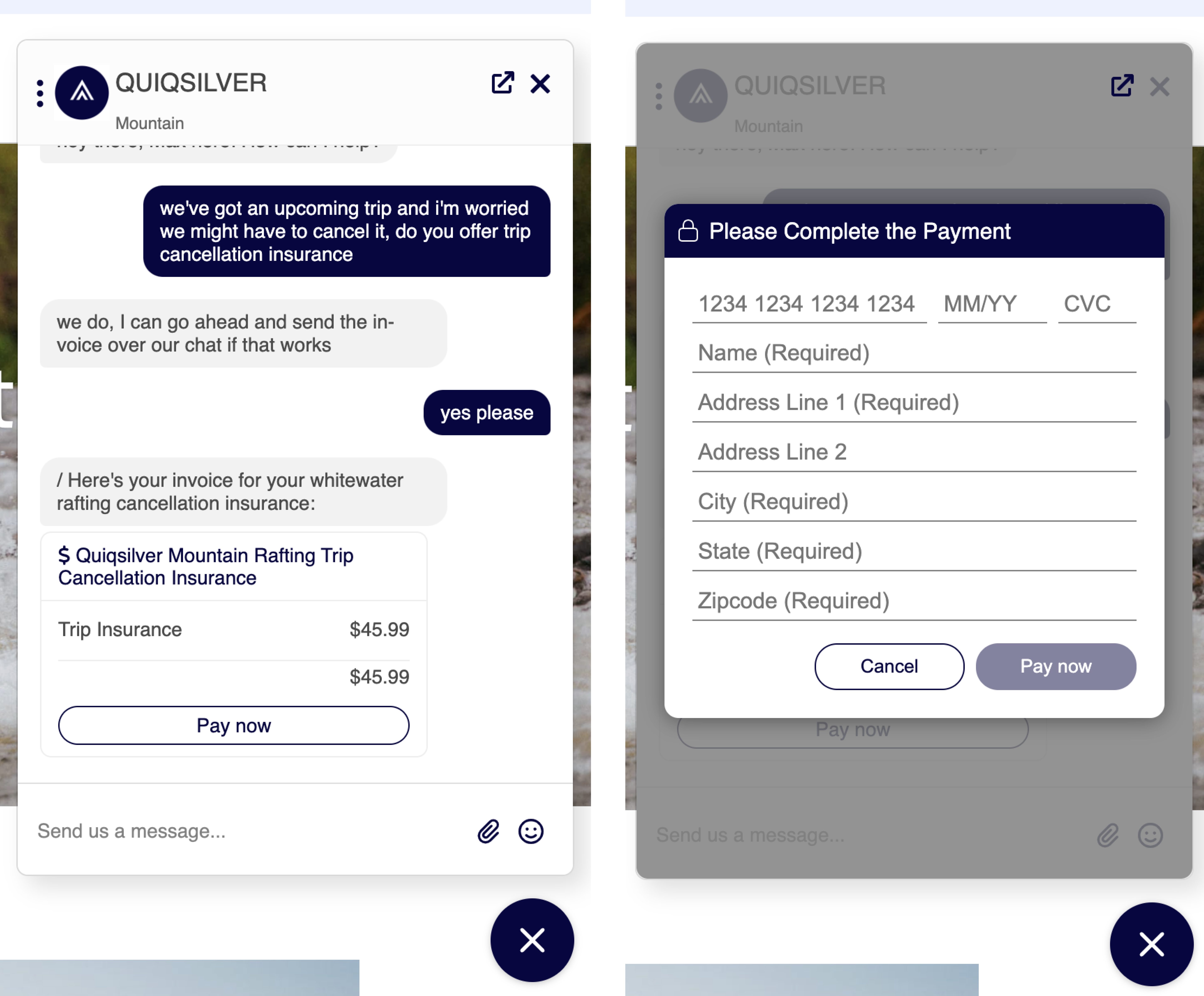
In the case of Web Chat and Apple Pay for Apple Messaging for Business, we have a native implementation of pay being used, which occurs right in the chat interface. For other channels, the user is taken to a secure pay screen and then returned to the conversation.
Hereâs what the same interaction looks like in Facebook Messenger:
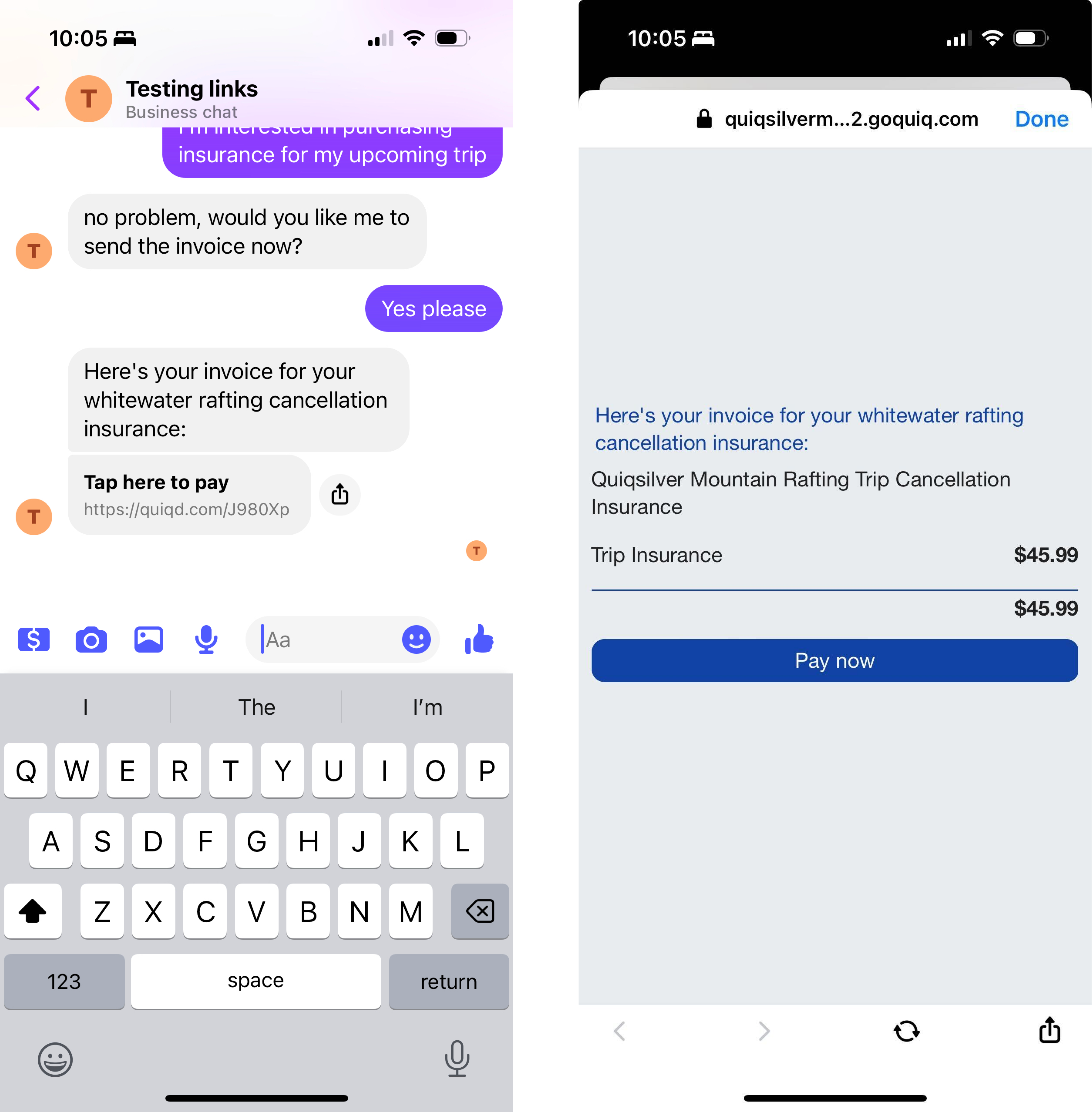
Regardless of what channel the pay message is being sent on, the user remains in a secure environment the entire time.
Proxy Interactions
Now, letâs say you want do something really fancy for a given platform, and donât care about having this interaction work on the other platforms. For example, what if you wanted to push an IOS app to a user in Apple Messages so that they could review product offerings or place an order? Is there a way to take advantage of the most powerful features a platform offers when appropriate to do so? Again the Quiq Conversational Engagement Platform solution has you covered. To accomplish this you would do the following:
{
"proxyInteractionâ: {
âinteractionTypeâ: âapple-interactive-messageâ,
âInteractionâ: {
âpayloadâ: <Apple Specific Payload to Push App>
}
},
âtranscriptHintsâ: {
âiconâ: {
âbuiltInIconâ: âdefaultâ
},
âheading1â: âPushed apple app to the userâ
}
}
Note that in this example we have specified a Proxy Interaction for Apple Messages. When you use this type of message, everything in the payload field is specific to each platformâs APIs, and is proxied along by Quiq, which allows you to make use of the most powerful features offered by the platform. Also note the âtranscriptHintsâ section. This allows you to specify how you want this type of message to be displayed to agents in the conversation transcript, and how you want it to be displayed anytime a textual representation of the conversation transcript is generated (email, copying to the clipboard, and CRM integrations). For specific details on the payloads accepted by each platform, please the API documentation for the platform in question.
Reply Directives
The Quiq Conversational Engagement Platform solution also offers the ability to tie workflow actions to user responses. This allows you to bind the responses to custom fields, or to route to certain queues based on the response. To demonstrate, letâs revisit the Quiq Reply example from earlier, where we presented the user with a list of options to describe their question. This time, letâs add the requirement that you want to set a custom field called âcase typeâ when they make a selection, and also route them to the right queue for handling their request. To accomplish this you would build the message as follows:
{
"text": "How can I help you today?",
"quiqReply": {
"replies": \[{
"text": "Account Info",
"directives": {
"fieldUpdates": [{
"field": "conversation.customFields.caseType",
"value": "AccountInfo"
}],
"newConversationRouting": {
"queueId": "accountQueue"
}
}
},
{
"text": "Service Request",
"directives": {
"fieldUpdates": [{
"field": "conversation.customFields.caseType",
"value": "ServiceRequest"
}],
"newConversationRouting": {
"queueId": "serviceQueue"
}
}
},
{
"text": "Product Question",
"directives": {
"fieldUpdates": [{
"field": "conversation.customFields.caseType",
"value": "ProductQuestion"
}],
"newConversationRouting": {
"queueId": "productQueue"
}
}
}
]
}
}
Note the new âreplyDirectivesâ section that is included on each response. This section allows you to specify actions that you want to be taken based on the userâs response. Here we are informing the system what fields to update, and in what queue to place the conversation. These are just a few examples of what you can do with Reply Directives. For a complete listing, please see the API documentation.
Reply Directives can currently be used within the Quiq Reply and Times Quiq Abstractions.
Combining Messages
Quiq Abstractions can also be combined to form richer more powerful messages. For example, you can combine the Card and Quiq Reply concepts to send out a product survey, and have the result saved off to a custom field:
{
"card": {
"title": âPlease rate your recently delivered product!â,
âsubTitleâ: âRustic Wood Entryway Benchâ,
âimageâ: {
âpublicUrlâ: âhttp://urlToPublicImageâ
},
âlinkâ: {
âurlâ: âhttps://linktonlinestoreâ
}
},
"quiqReply": {
"replies": [{
"text": "Bad!",
"directives": {
"fieldUpdates": [{
"field": "conversation.customFields.satisfactionScore",
"value": "1"
}]
}
},
{
"text": "Ok",
"directives": {
"fieldUpdates": [{
"field": "conversation.customFields.satisfactionScore",
"value": "5"
}]
}
},
{
"text": "Awesome!",
"directives": {
"fieldUpdates": [{
"field": "conversation.customFields.satisfactionScore",
"value": "10"
}]
}
}
]
}
}
In this example Quiq Abstractions will be combined into a single message if the platform supports doing so, or multiple messages if not. The Quiq Interaction App will also be leveraged when appropriate so that users can see the entire combined message when necessary.
Google RBM and Webchat:
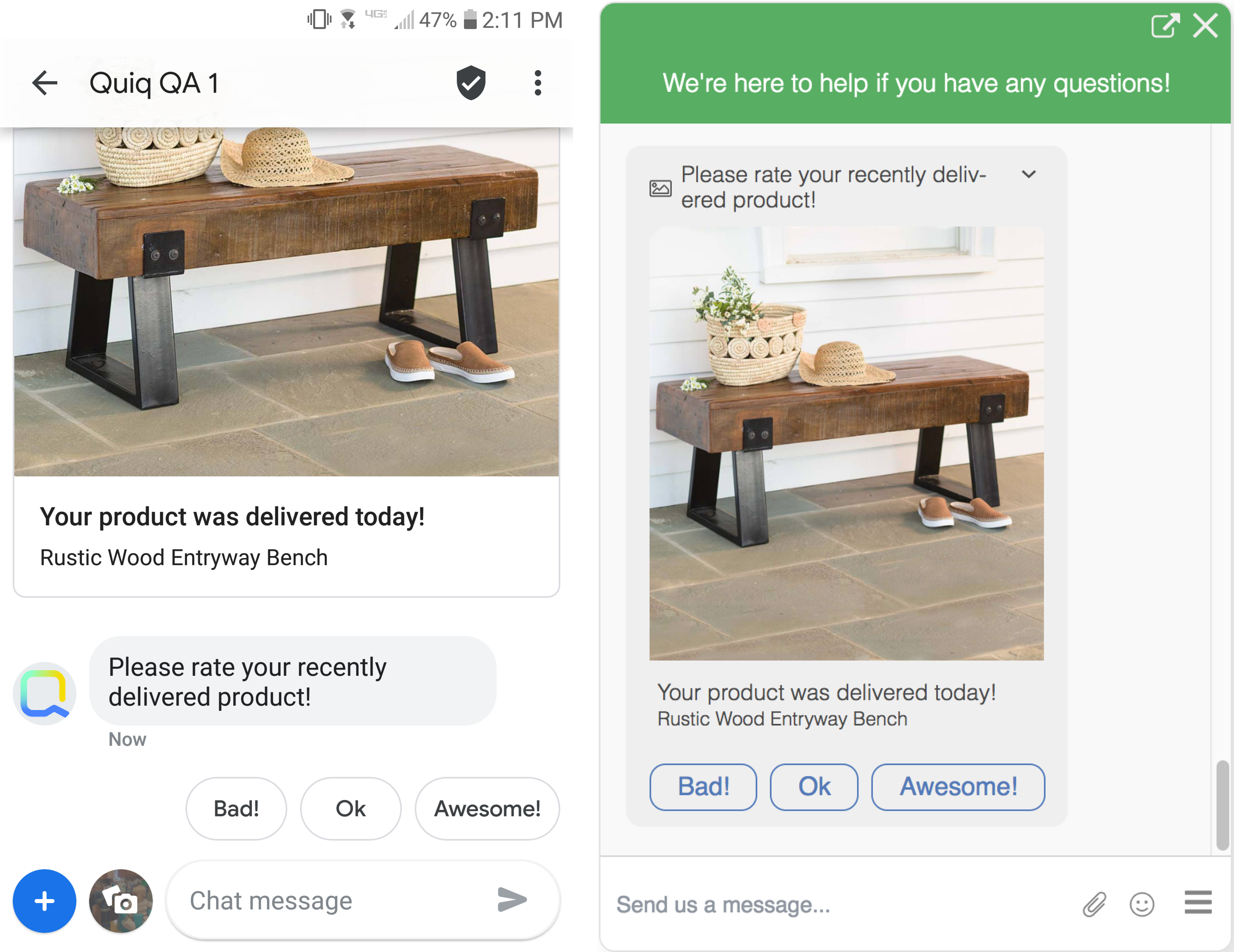
Apple Messages:
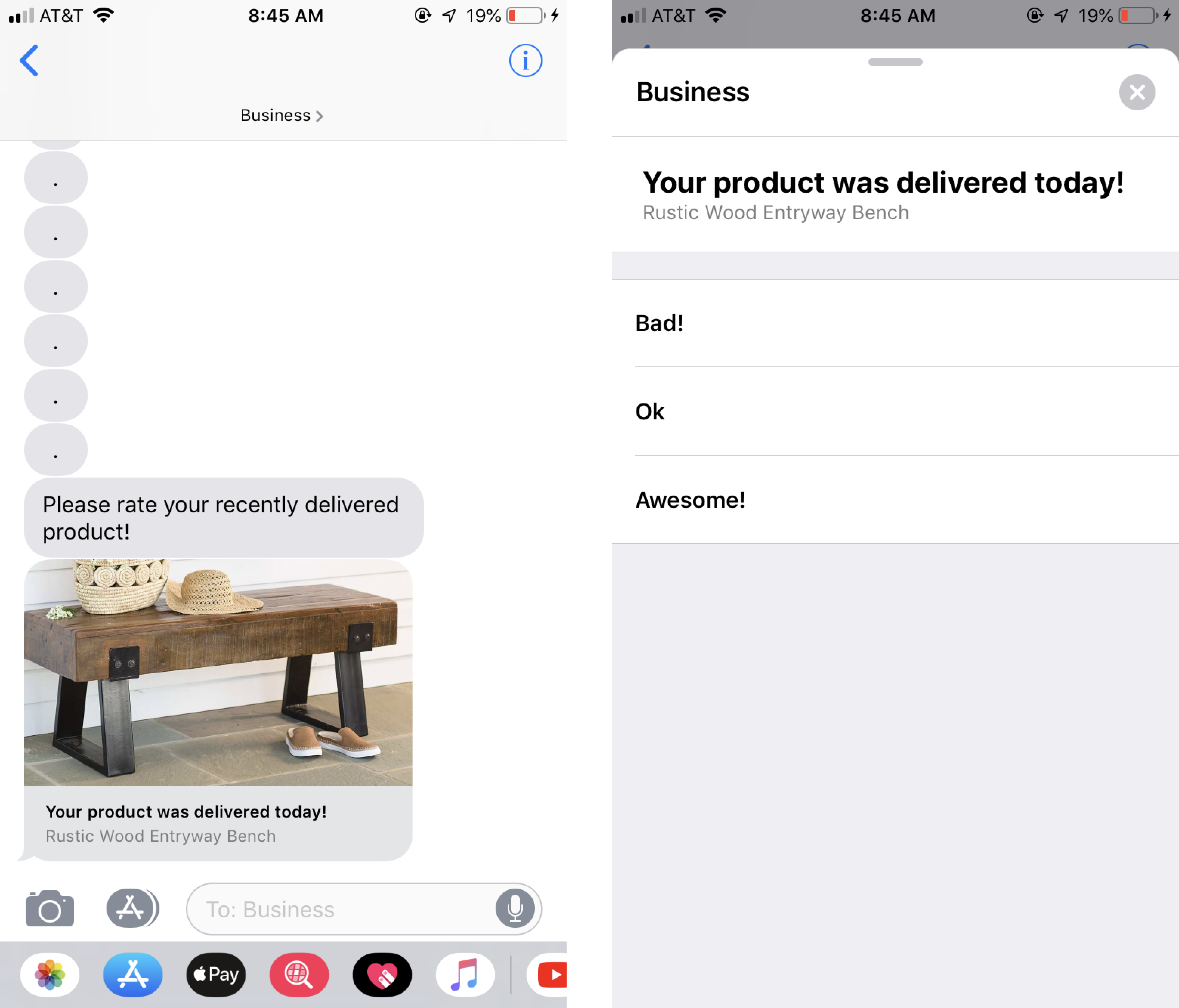
This is what the combined message would look like in SMS with the Quiq Interaction that will be displayed if the user wants to see the entire message all together and taps the shortened link.
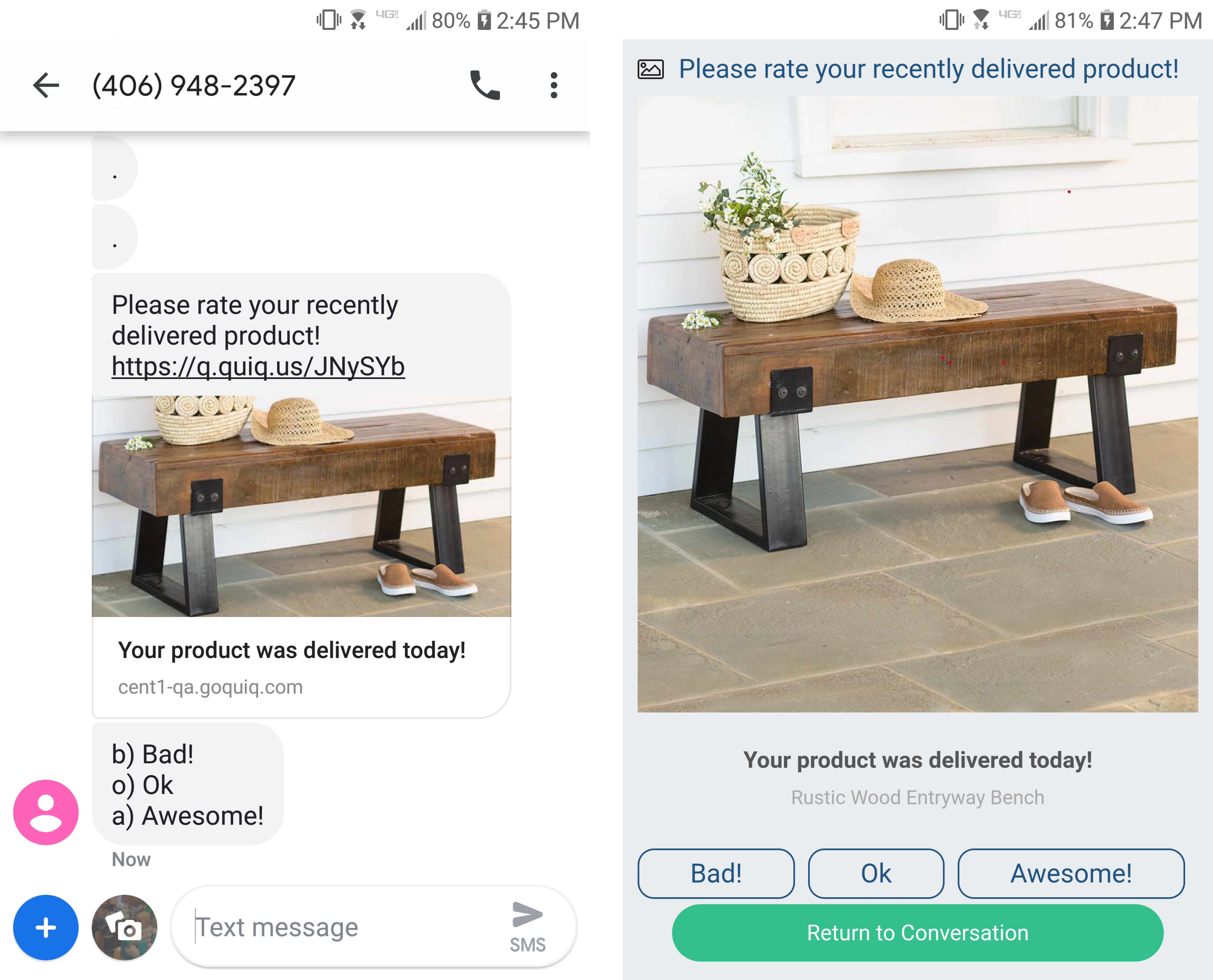
Updated 24 days ago